I’m now on my holidays in Bali, which should explain lack of updates on my blog. It is rainy season here, thus the weather is quite cloudy and rains at least once a day. I personally think this type of weather is pretty much perfect for holidays, as it is not too cold and not too hot.
Thursday, January 26, 2006
Posted @ at
8:27 pm
What do you think? (there
are 3
comments)
Labels: bali, bali photos
Saturday, January 14, 2006
In the part one of the article series I’ve introduced the very basics of mobile application development that is a preparation of mobile application development environment. I’ve also introduced the technology that will be used in all the series of “Mobile Application Development: Can I do it?”. The chosen technology is Java 2 Platform, Micro Edition (J2ME) and NetBeans IDE (Integrated Development Environment) as our development tool. Both products are FREE for commercial and non-commercial use allowing everyone to start mobile development immediately without spending a single dollar. After reading the part one of the article series, many of you tried the sample projects provided together with NetBeans 4.1. Base on the initial input I've received it seems that many of you got surprised with impressive results that can be achieved with only short Java code. You also start asking “How to make my own J2ME project using NetBeans?” or “How to transfer builded application to my mobile phone?”. The second part of the article series provides step-by-step answer to both questions.
In the next four parts of the series I’d like to focus on the fun part of a mobile application development which is making mobile games. I’ll show how to build a simple, yet interesting game step-by step, from very basic code, graphics up to the code optimisation and fully working game.
Let’s start
The very early stage of mobile game development is usually a creation of a very basic template (skeleton) type of our game code. Such skeleton code includes the basic functions of our mobile game such a handling graphics, control of the game by the users, control of application lifecycle. Thus, in our earliest J2ME game coding we will create our first MIDlet – that’s how we name Java 2 program on embedded devices.
In the first step let’s create new project in NetBeans IDE. Start the NetBeans IDE and select File -> New Project (or use keyboard shortcut Ctrl+Shit+N). A New Project wizard will appear.In the first choice we have to choose the type of project. The best option for creating a mobile game skeleton project is to select
In the next menu set the name for the project i.e. MyFirstGame and the location of the project where all project files will be stored. For the purpose of this article please do not select the “Create Hello MIDlet” option, as we will create MIDlet ourselves. Click Next.
The important options to configure at this stage are Device Configuration and Device Profile.
In the first Device Configuration setting the CLDC stands for Connected, Limited Device Configuration. In general it is part of framework that provides the most basic set of libraries and a virtual-machine feature that allows our MIDlet to run on the device. It is designed for resource-constrained devices such as smart phones, PDAs and small electronic equipment. There are currently two CLDC specifications available, version 1.1. (JSR 139) and the first release version 1.0 (JSR 30). At this stage you need to check which version is supported by mobile phone that you want to use in application development. My device is Sony-Ericsson P900 and by referring to the documentation I checked that it supports CLDC version 1.0. Thus, my option for Device Configuration is CLDC-1.0.
Second important setting is a Device Profile, available in two options MIDP-1.0 and MIDP-2.0. MIDP stands for Mobile Information Device Profile, and is also part of the J2ME framework, that together with CLDC enables Java on your mobile phone. By referring to documentation I checked that my SE-P900 phone supports MIDP-2.0 thus this will be my option. MIDP-2.0 provides lots of new and very useful features, which will make our game development much easier. Unfortunately, if your mobile phone does not support the new MIDP-2.0, some parts of the code provided in this article may not be supported on your mobile phone (especially GameCanvas related). You will be able to work on your mobile application in NetBeans but it will be a problem to make it work on your MIDP-1.0 phone.
After clicking Finish, MyFirstGame project will be ready for development.
Figure 3. Creation of New MIDlet.
As previously we decided not to create default MIDlet, we need to create it manually. Thanks to NetBeans, it is extremely simple. Simply click right button on (
package mygame; import javax.microedition.midlet.*; import javax.microedition.lcdui.*; public class MyGameMIDlet extends MIDlet { public void startApp() { } public void pauseApp() { } public void destroyApp(boolean unconditional) { } }
The first method startApp() is called by Application Management Software (AMS) when MIDlet starts or it is being resumed. Since it is invoked after resume, it is clear that it can be executed more than once. For this reason it is important to control how new objects are initialised in this part of the code, as there is a risk of initialising object more than once.
The next mandatory method is pauseApp(). It is invoked by AMS in situation such as user receiving a phone call during the game, low battery warning etc. and can be used to minimise CPU, memory or even a power consumption, when application is not in the active state. It is important to remember that pauseApp() is not always called when MIDlet is going into background (loose focus), as it is on Series 60 phones. In such situation MIDlet should detect when application is not in focus and call notifyPaused() method.
The last mandatory method is destroyApp(). This method is called to destroy MIDlet by AMS to prepare MIDlet for termination. It should execute clean-up actions releasing resources etc. and notify AMS about completion of cleanup tasks with notifyDestroyed().
These three methods are part of something called MIDP Lifecycle. It is very important for every J2ME programmer to know this subject well, thus I refer to the PDF document Programming the MIDP Lifecycle on Symbian OS that explains it in details and provides information on mistakes that should be avoided by newbie J2ME programmers. I also strongly recommend reading MIDlet Lifecycle Article on Sun Developers web-site.
Base on information mentioned in these guidelines, our implementation of MIDP lifecycle in the MyGameMIDlet.java looks as follow:package mygame; import javax.microedition.midlet.*; import javax.microedition.lcdui.*; public class MyGameMIDlet extends MIDlet { private final static int PAUSED = 0; private final static int ACTIVE = 1; private final static int DESTROYED = 2; private int stateTracker = PAUSED; public void startApp() { stateTracker = ACTIVE; } public void pauseApp() { stateTracker = PAUSED; notifyPaused(); } private synchronized void performDestroyCleanup() { if (stateTracker != DESTROYED) { stateTracker = DESTROYED; notifyDestroyed(); } } public void destroyApp(boolean unconditional) throws MIDletStateChangeException { performDestroyCleanup(); } }
Beside MIDlet, one more Java Class will be required, a GameCanvas. This is a special class that will handle game-specific features such as efficient graphics or game control with keys pressed by the user. For those one unfamiliar with GameCanvas I recommend to read the Game Canvas Basics article followed by Using GameCanvas in MIDP2.0.
We can add this class to our project by right-clicking on the package (mygame or
import javax.microedition.lcdui.Graphics; import javax.microedition.lcdui.Font; import javax.microedition.lcdui.game.GameCanvas;
Their functions are as follow: javax.microedition.lcdui.Graphics (provides simple 2D geometric rendering capability); javax.microedition.lcdui.Font (provides fonts capability); javax.microedition.lcdui.game.GameCanvas (introduced in MIDP-2.0, provides gaming features).
public class MyGameCanvas
as follow:
public class MyGameCanvas extends GameCanvas implements Runnable {Use of GameCanvas, also requires us of super() to suppress the normal key event mechanism, inherited from the
Canvas
class, as we do not require these notifications in our MyGameCanvas. Finally, implementation of Runnable interface for the purpose of tasks (read more here) requires us to add one more method called run(). The modified basic MyGameCanvas.java code looks as follow:
package mygame; import javax.microedition.lcdui.Graphics; import javax.microedition.lcdui.Font; import javax.microedition.lcdui.game.GameCanvas; public class MyGameCanvas extends GameCanvas implements Runnable { public MyGameCanvas() { super(true); } public void run() { } }
Game loop
Every game is build with the same concept of repetitively executed code that modifies graphics, resulting in animation and game action. Thus, in next step we need to create a primary game loop.
Such game loop will require creation of a new thread, starting the thread, within the thread executing all the actions and repating these actions with specified time delay. To organise the code better, we also added custom startGame() and stopGame() methods. The startGame() method will be used to execute the thread, which will then call run() and go into the game loop. The stopGame() method will be used to stop the thread. The delay between repeating tasks in the loop can be easily done with Thread.sleep(milisecond), which will sleep a thread for a specified number of milliseconds, before repating the actions and creating a next frame of the game.The modified MyGameCanvas.java code looks as follow:
package mygame; import javax.microedition.lcdui.Graphics; import javax.microedition.lcdui.Font; import javax.microedition.lcdui.game.GameCanvas; public class MyGameCanvas extends GameCanvas implements Runnable { public long GameFrameDelay = 30; Thread thread = new Thread(this); public MyGameCanvas() { super(true); } public void startGame() { thread.start(); } public void stopGame() { thread = null; } public void run() { while ( true ) { try { Thread.sleep(GameFrameDelay); } catch (InterruptedException ie) { stopGame(); } } } }
At this stage we have finished our game loop. However, if we run (press F6) the MIDlet, we will not see any game canvas or any graphics. You probably wonder why? The answer is very simple, it is because we haven’t linked MyGameCanvas with MyGameMIDlet. To do it we need to start MyGameCanvas thread from MyGameMIDlet and link the canvas.
package mygame; import javax.microedition.midlet.*; import javax.microedition.lcdui.*; public class MyGameMIDlet extends MIDlet { private final static int PAUSED = 0; private final static int ACTIVE = 1; private final static int DESTROYED = 2; private int stateTracker = PAUSED; private Display mygameDisplay; private MyGameCanvas mygameCanvas; public void startApp() { if (mygameCanvas == null) { mygameCanvas = new MyGameCanvas(); mygameCanvas.startGame(); } mygameDisplay.getDisplay(this).setCurrent(mygameCanvas); stateTracker = ACTIVE; } public void pauseApp() { stateTracker = PAUSED; notifyPaused(); } private synchronized void performDestroyCleanup() { if (stateTracker != DESTROYED) { stateTracker = DESTROYED; notifyDestroyed(); } } public void destroyApp(boolean unconditional) throws MIDletStateChangeException { performDestroyCleanup(); } }
If we run our project in the emulator (F6), it will only produce a blank screen. This is simple because we haven’t added any graphic to MyGameCanvas code, yet.
Adding Graphics to MyGameCanvas
Now, let’s try to make our application display some graphics during the game loop. The beginning step would be creating a background for our graphics. To keep it simple we will fill up the screen with one solid colour. The simplest method to do so is to create a coloured rectangle that fits the size of the screen. To create such rectangle, we need to first create an object that will be used for graphic operations using getGraphics() method. As next step, we need to get the width and height of the mobile device screen using getWidth() and getHeight(). With this information we know dimension of the rectangle required to cover all the space of the screen. Now only need to set the drawing colour using Graphics method setColor() and fill the canvas with rectangle using fillRect() method, with a dimensions set to cover the screen.
The rectangle is created on game canvas. However, if you run the project the rectangle will not appear on the mobile device screen yet. To do so we still need to flush all the graphics changes on the screen. For this purpose we can create a custom method called paintScreen() (name it whatever you like) that calls flushGraphics() method, resulting in graphic changes appearing on the screen.
The modified part of the MyGameCanvas.java code looks as follow:
... public void run() { Graphics g = getGraphics(); int scrWidth = getWidth (); int scrHeight = getHeight(); while ( true ) { g.setColor(0x00ff00); g.fillRect(0, 0, scrWidth, scrHeight); paintScreen(g); try { Thread.sleep(GameFrameDelay); } catch (InterruptedException ie) { stopGame(); } } } protected void paintScreen(Graphics g) { flushGraphics(); } ...If we run the MIDlet a green (0x00ff00 in hex) background should appear on the screen. After checking it you will probably quickly notice one problem that is lack of ability to close midlet by the user. For this purpose we need to add Exit command to our game canvas. This will require implementation of CommandListener, creation of a new exitCommand command and adding command to the mygameCanvas with addCommand method. Additionaly, we will also need to create commandAction()method that will handle command actions. Additionally, we need to make a small change to startApp() to provide us with control on the state change exceptions. Modified MyGameMIDlet.java looks as follow:
package mygame;
import javax.microedition.midlet.*;
import javax.microedition.lcdui.*;
public class MyGameMIDlet extends MIDlet
implements CommandListener {
private final static int PAUSED = 0;
private final static int ACTIVE = 1;
private final static int DESTROYED = 2;
private int stateTracker = PAUSED;
Command exitCommand = new Command("Exit",Command.EXIT,2);
private Display mygameDisplay;
private MyGameCanvas mygameCanvas;
public void startApp()throws MIDletStateChangeException {
if (mygameCanvas == null) {
mygameCanvas = new MyGameCanvas();
mygameCanvas.startGame();
mygameCanvas.addCommand(exitCommand);
mygameCanvas.setCommandListener(this);
}
mygameDisplay.getDisplay(this).setCurrent(mygameCanvas);
stateTracker = ACTIVE;
}
public void pauseApp() {
stateTracker = PAUSED;
notifyPaused();
}
private synchronized void performDestroyCleanup() {
if (stateTracker != DESTROYED) {
stateTracker = DESTROYED;
notifyDestroyed();
}
}
public void destroyApp(boolean unconditional)
throws MIDletStateChangeException {
performDestroyCleanup();
}
public void commandAction(Command c, Displayable s) {
if (c.getCommandType() == Command.EXIT) {
try {
destroyApp(true);
} catch (MIDletStateChangeException ex){ }
}
}
}
Keypad based game control
package mygame; import javax.microedition.lcdui.Graphics; import javax.microedition.lcdui.Font; import javax.microedition.lcdui.game.GameCanvas; public class MyGameCanvas extends GameCanvas implements Runnable { public long GameFrameDelay = 30; String txt = new String("Hello. Press Keys."); Thread thread = new Thread(this); public MyGameCanvas() { super(true); } public void startGame() { thread.start(); } public void stopGame() { thread = null; } private void processKeyStates() { int keyState = getKeyStates(); if ((keyState & UP_PRESSED) != 0) { txt = "Key: UP"; } if ((keyState & DOWN_PRESSED) != 0) { txt = "Key: DOWN"; } if ((keyState & LEFT_PRESSED) != 0) { txt = "Key: LEFT"; } if ((keyState & RIGHT_PRESSED) != 0) { txt = "Key: RIGHT"; } if ((keyState & FIRE_PRESSED) != 0) { txt = "Key: FIRE"; } } public void run() { Graphics g = getGraphics(); int scrWidth = getWidth (); int scrHeight = getHeight(); Font myfont = g.getFont(); myfont = Font.getFont(Font.FACE_SYSTEM, Font.STYLE_BOLD, Font.SIZE_MEDIUM); int fontHeight = myfont.getHeight(); int fontWidth = myfont.stringWidth(txt); g.setFont(myfont); while ( true ) { g.setColor(0x00ff00); g.fillRect(0, 0, scrWidth, scrHeight); processKeyStates(); g.setColor(0x990000); fontWidth = myfont.stringWidth(txt); g.drawString(txt, (scrWidth - fontWidth)/2, (scrHeight - fontHeight)/2, g.TOP|g.LEFT); paintScreen(g); try { Thread.sleep(GameFrameDelay); } catch (InterruptedException ie) { stopGame(); } } } protected void paintScreen(Graphics g) { flushGraphics(); } }
The result of running the final code in the emulator is a green background with welcome text changing base on the user input, as seen below:
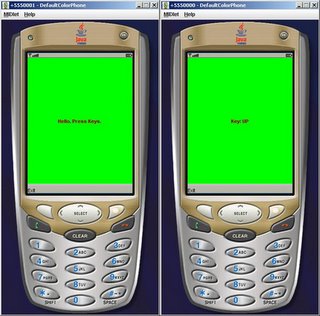
Figure 5. Final template running in emulator.
Now, the only task left is to Clean and Build Main Project (Shift + F11) [Figure ].
The generated file MyFirstGame.jar can be uploaded to the mobile phone via Bluetooth, installed and launched. Below screenshot shows MIDlet running on my SE-P900 phone.
Conclusion
Thanks to NetBeans IDE and J2ME technology, mobile game development is trivial. With less than 100 lines of Java code we were able to create a MIDlet that displays text and graphics, can be controlled from mobile phone keypad and integrates with phone Application Management Software (AMS). We were also able to quickly verify the result thanks to emulator, which can be launched from NetBeans with a click of a button. Finally, with another click of the button we were able to clean the code, build it and run the generated JAR file on a mobile phone. Clearly, creating a basic mobile game is not as difficult as you may previously have thought.
Posted @ at
1:29 pm
What do you think? (there
are 13
comments)
Thursday, January 12, 2006
Indcoup writes about problems of food safety in Jakarta. Dangerous food is on my top 3 most disturbing things in Jakarta, just after the nightmare traffic and scary butcher doctors. Just read the post and join the discussion. Meanwhile, Jakartass writes about various problems with customer service often faced by ppl in Jakarta. All very interesting read.
Posted @ at
5:36 pm
What do you think? (there
are 0
comments)
Wednesday, January 11, 2006
A friend of mine has been researching Windows Embedded Open Type (EOT) Font vulnerability that he discovered in Windows about a week ago. Just when he finished the analysis, eEye released the vulnerability advisory, thus being ahead of him. Anyway, to this moment his analysis is the best known publicly available analysis of this vulnerability.
Overall, the vulnerability should be considered as CRITICAL, mostly due to the simple fact that EOT is supported by Internet Explorer, Microsoft Word, Excel and several other applications that allow to include EOT fonts in the document/file. It means that every .doc file or Internet web-site could potentially result in malicious application being exedcuted on your computer. Thus, it is extremely important to update all Windows computers at office or home quickly. You can download patch from here. Install it ASAP!
Posted @ at
4:22 am
What do you think? (there
are 0
comments)
Tuesday, January 10, 2006
I’ve received number of interesting e-mails from my readers in relation to the Wi-Fi Hotspots in
English version:
2. Due to security reasons, Customers are advise not to use Hotspot for electronic transaction or other confidential information.
4. Hereby the Customer understands and aware of any risk on using Hotspot, therefore Customer releases CBN for any loss or responsibility due to such risk.
Indonesian version:
2. Karena alasan keamanan, Pelanggan tidak dianjurkan menggunakan fasilitas Hotspot untuk melakukan transaksi keuangan atau informasi rahasia lainnya.
4. Pelanggan dengan ini memahami segala resiko atas penggunaan atau ketidaksanggupan layanan yang disediakan, untuk itu Pelanggan bersedia membebaskan CBN dari segala tuntutan ganti rugi maupun tanggung jawab yang timbul akibat resiko sebagaimana telah dijelaskan.
I verified correctness of this information and as you can see on the screenshot below, the CBN Hotspot Terms of Service indeed include such clauses:
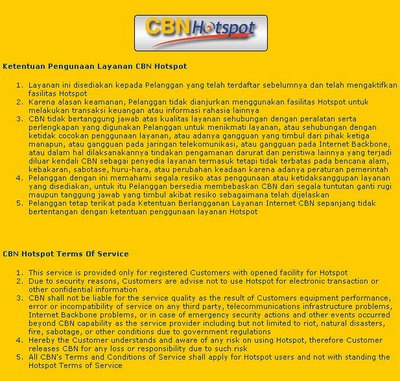
When reading these terms and conditions set by CBN, several question arise. First of all, what happens if I use the insecure hotspot service to receive my e-mail and some hacker benefiting on lack of encryption of wireless data and captures my e-mail password and reads my confidential data? The terms of service do not mention anything about lack of encryption of service, it only states Customer understands and aware of any risk on using Hotspot, therefore Customer releases CBN for any loss or responsibility due to such risk, plus the article about on-line banking and confidential information. I don’t know how about my readers, but “any risk” sounds very much broad to me. If presumably there is a problem with billing on the hotspot and the consumer bill for use of the service increase to a very high amount, is it also included in any risk? I say there is some risk of damage to my wireless card, thus how about if my wireless card gets damaged due to malfunction of wireless hotspot? Is it also within any risk? Stating that company is not responsible for any risk sounds totally crazy to me.
I couldn’t believe that offering a commercial telecommunication service to consumers with such terms is legal. Unfortunately, I can not answer this question myself as my understanding of Indonesian law is very blurry. I also don’t have a license of an Indonesian lawyer allowing me to provide any legal opinion, so to clarify everything I decided to seek opinion of experts in consumer protection and telecommunication laws. My choice was REM Asia Pacific & Co. law firm as they got one of the best legal experts in both telecommunication and consumer protection laws, which perfectly fits my need.
The opinion of REM Asia Pacific’s Partner, Reno Iskandarsyah, is as follow:
Is it legal? The answer is, No! In
The Terms and Conditions given by CBN, is categorized as “standard clause”. It contains the rights of CBN (as service provider) and the obligations of consumer (as subscriber). However, as obviously noticed, the positions of the service provider and the subscriber are not equal in CBN case. A service provider shall not simply leave an option to its subscriber, i.e.: if you disagree with the terms, you can leave CBN and no longer using their network, if the terms are acceptable to you, you may continue using the network, but, when the network disturbance occures, CBN shall not be responsible for any loss incurred.
Under Article 4 Act No.8/1999 consumer has the right to enjoy comfort, protection, safety, while using or consuming goods or services, and she/he has a right to be compensated for any malfunction. Additionally, Article 7 obliged the service provider to provide the service in good faith, in truth (regarding giving information about the service), honesty, non- discrimination, as well as giving warranty for the standard quality of the service or goods.
The issue of “standard clause” in CBN Terms and Conditions, basically in breach of Article 18. Under this article, a company may not or not allowed to create a standard clause in every documents nor terms and conditions regarding:
a. assignment of liability of the company;
b. consumer is abide by the amendment to the standard clauses made by the company during the utilization or consumption of the purchased service or good.
A breach of this article will be subject to a crime sanction of 5 (five) years in jail or the penalty of Rp. 2,000,000,000 (IDR Two Billion, ~US$210,000).
Clearly, in
Frankly, I feel completely stunned with this answer! Considering the simple fact that
I’ve also asked Rakhmayanti Esther Makainas – well known expert in telecommunication law – for her comment on this matter. Here it goes:
In
After reading opinion of two highly qualified lawyers, I think you already know what to do in case of problems with your ISP.
From the technical point of view, I clearly understand that providing insecure, unencrypted wireless Internet access service creates a great risk to the consumer. It doesn't take much hacking skills to sit down at a café with hotspot and begin intercepting and reading all the unencrypted wireless transmissions passing through the air. There are number of ways to secure public wireless hotspot and it seems that ISP simply doesn't bother to implement it. It also does not involve high cost, as the wireless service can be effectively secured with a simple solution.
FYI in
I'd like to end this article with a quotation from CBN's web-site expressing the vision of the company:
As the leader among Internet Service Providers in Indonesia, CBN is working for a recognition as“The Brand You can Trust”.
Trust?! I leave comment on that to the growing number of readers of my Blog.
PS: If you are frustrated with service offered by your ISP, drop me an e-mail.
Posted @ at
4:09 pm
What do you think? (there
are 4
comments)
Labels: indonesian law, wifi
Saturday, January 07, 2006
As I work in Indonesia/Singapore as an IT consultant and auditor (mainly IS Security), I've gotten used to receiving a number of interesting IT related questions from my clients and friends. As I want to run a few regular sections on my Blog, I had an idea of a regular section that might be very useful to many. It is simply called "Ask Marek", where I will try to answer on all possible IT related questions from my readers. The idea certainly comes from The Slashdot. However, in difference to Slashdot, the answer here will be provided not by readers commenting on the post, but by me. However, feel free to post your own opinions in comments section. FYI I can not answer on all the questions I receive, yet I promise I'll try to answer on at least one question a week (unless I'm on holidays).
I received a very interesting question from Michael: How to set-up Internet connection for my SME office in Jakarta? Thanks Michael for this question. (Malcolm, I hope part of this article will answer on your question related to getting relatively cheap and efficient Internet connectivity in Jakarta; Volker, I also hope this will explain your question of connecting your office to Internet). In a typical SME (Small/Medium Enterprise) office with less than 50 employees, normally the following Internet related elements will be required to support your business:- Internet connectivity,
- Company’s e-mail access,
- Company’s web-site.
- first of all, office buildings where ISPs have their NOC (Network Operation Centres),
- office buildings where ISPs have their office,
- office buildings near location where ISPs have their NOC – best if you can see the ISP office from your window.
- PHP,
- web-statistics,
- mySQL database.
- e-mail forwarding and aliases,
- spam filters,
- anti-virus.
- Get cheap web and mail hosting in Europe together with domain – make sure hosting company provides e-mail forwarding service, (my hosting recommendation is www.creativenet.de),
- Upload your web-site to the server,
- Open Gmail accounts for all employees,
- For each employee set-up e-mail forwarding at hosting server to forward e-mails to Gmail accounts. For example forward some.employee@yourcompany.com to some.employee@gmail.com.
- Ask your employees to use Gmail web-interface or configure their favourite e-mail client to use Gmail.
Posted @ at
1:30 am
What do you think? (there
are 5
comments)
Thursday, January 05, 2006
Modern mobile phones provide number of advanced functions, among which possibility of running mobile applications seems to be the most interesting feature. It changes a standard mobile phone into a mini-size yet still powerful computer able to run various applications. Available mobile applications range from games or and organizer type of applications to advanced business applications designed for company’s portable employees. Moreover, the number of mobile application developers is rapidly growing and year by year the developed applications are better, faster and more innovative. However in difference to international trend, the technology of Symbian OS and J2ME development is very far from being popular in Indonesian. It is extremely difficult to find local application developers experienced in J2ME and unfortunately mobile application development industry is almost unexisting here. In a hope of creating more interest in the area of mobile application development within Indonesian application developer community, I prepared a series of articles on J2ME development articles. In this first part of the series of articles, I introduce the very basis of mobile application development, which is setting-up mobile application development environment on your workstation.
Mobile applications for Symbian OS based mobile phones can be developed in various programming languages. The most popular programming language on mobile phones is definitely Java. This programming language named after a cup of Javanese coffee, provides simple but truly powerful mean of building portable applications. The great popularity of Java on mobile phones comes mostly from the wide support of major mobile phone manufacturers for the J2ME (Java 2 Mobile Edition) applications and great support by major corporations such as Sun Microsystems and Nokia. Furthermor, the overall idea of Java multi-platform compatibility makes it a perfect choice for portable devices such as mobile phones. One of the leading Java development tools on the market is NetBeans product. In June 2000 NetBeans was generously made open source by Sun Microsystems, which still remains the project sponsor. NetBeans is an open-source project that liberated mobile application development and provided strong alternative to commercial mobile application development enviroments. It allowed to replace a complicated process of configuring development environment that discouraged number of users from trying development of J2ME applications, into a simple, trivial to install and powerful mobile application development tool. What previously took hours now takes minutes and provides excellent comfort for developers. The NetBeans project is also a community where people from just about any country, have the ability to make a wide variety of contributions and exchange their knowledge. The two base NetBeans products are NetBeans IDE and NetBeans Platform. Thanks to Sun Microsystems, both products are free for commercial and noncommercial use, which makes it an excellent tool for beginners in a mobile application development. For this reason I decided to focus my article on the use of NetBeans IDE application. Since NetBeans is free, you can start developing applications from now without any adiditonal cost, and if you are commited you can even make profit out of it! Let’s start The first stage of mobile application development is getting all the necessary applications. As previously mentioned everything we need can be freely downloaded from the Internet. The list of basic elements of our application development enviroment goes as follow:- J2SE(TM) Development Kit,
- NetBeans IDE,
- NetBeans Mobility Pack Installer.
- jdk-1_5_0_06-windows-i586-p.exe,
- netbeans-4_1-windows.exe,
- netbeans_mobility-4_1-win.exe,
Posted @ at
2:28 am
What do you think? (there
are 5
comments)
Monday, January 02, 2006
Refering to my previous post on Mobile Viruses in Jakarta :-)
Posted @ at
5:52 pm
What do you think? (there
is 1 comments)
Sunday, January 01, 2006
My Blog has been mentioned in the Jakartass Blog Award 2005 :-) Thanks Jakartass and keep up the good work of independent journalist!
Posted @ at
9:56 pm
What do you think? (there
are 0
comments)
Happy New Year, Selamat Tahun Baru, Xin Nian Kuai Le!
My best Happy New Year Wishes to you all! Let this New Year 2006 (11111010110) be prosperous for us all. But that’s not the end yet. For the New Year eve I’ve prepared a small and special geek style gift for all of you. My gift is a calendar of public holidays that you can import to your Microsoft Outlook or other electronic organizer. I hope this will help you to remember about holidays and you will always remember to wish everything the best to all your friends in various countries around :-) I hope four hours of my work to put all this together was useful and you will find this little gift useful. I prepared lists of Public Holidays inTo import it into Microsoft Outlook, simply open Microsoft Outlook Calendar and click: File -> Import and Export. Select Import an iCalendar or vCalendar file (.vcs) from the list and click Next. Now change the "Files of type" to "vCalendar Format (*.vcs)" and locate the downloaded .VCS file, click OK to import. The events will successfully import into the Outlook as events 7am-8am or 7am-6pm (depending on the version you downloaded). If you wish to import it to your mobile phone, simply synchronise your phone with Outlook after importing events from .VCS file.
Good luck and once again Happy New Year 2006!
PS: If you like my calendar, please send link to this Happy New Year site to your friends.
Following is the list of Public Holidays in
10.01.2006 - Idul Adha 1426H - PH in ID
29.01.2006 - Chinese New Year 2557 - PH in ID
31.01.2006 - Islamic New Year 1427H - PH in ID
30.03.2006 - Hari Raya Nyepi 1928 - Balinese Hindu New Year - PH in ID
11.04.2006 - Prophet Muhammad's Birthday - PH in ID
14.04.2006 - Good Friday - PH in ID
13.05.2006 - Hari Raya Waisak - PH in ID
25.05.2006 - Ascension Day - PH in ID
17.08.2006 - Indonesian Independence Day - PH in ID
21.08.2006 - Isra Mi'raj Prophet Mohammad SAW - PH in ID
23.10.2006 - Cuti Bersama - PH in ID
24.10.2006 - Idul Fitri 1427 H - PH in ID
25.10.2006 - Idul Fitri 1427 H - PH in ID
26.10.2006 - Cuti Bersama - PH in ID
27.10.2006 - Cuti Bersama - PH in ID
25.12.2006 - Christmas Day - PH in ID
31.12.2006 - Idul Adha 1427H - PH in ID
Total: 17 days
10.01.2006 - Hari Raya Qurban - PH in MY
29.01.2006 - Chinese New Year - PH in MY
30.01.2006 - Chinese New Year - PH in MY
31.01.2006 - Awal Muharam (Maal Hijrah) - PH in MY
11.04.2006 - Prophet's Muhammad's Birthday - PH in MY
01.05.2006 - Labour Day - PH in MY
12.05.2006 - Wesak Day - PH in MY
03.06.2006 - Birthday of SPB Yang di-Pertuan Agong - PH in MY
31.08.2006 -
21.10.2006 - Deepvali - PH in MY
24.10.2006 - Hari Raya Puasa - PH in MY
25.10.2006 - Hari Raya Puasa - PH in MY
25.12.2006 - Christmas Day - PH in MY
31.12.2006 - Hari Raya Qurban - PH in MY
Total: 14 days
10.01.2006 - Hari Raya Haji - PH in SG
29.01.2006 - Chinese New Year - PH in SG
30.01.2006 - Chinese New Year - PH in SG
31.01.2006 - Islamic New Year 1427H - PH in SG
14.04.2006 - Good Friday - PH in SG
01.05.2006 - Labour Day - PH in SG
12.05.2006 - Vasak Day - PH in SG
09.08.2006 -
21.10.2006 - Deepavali - PH in SG
24.10.2006 - Hari Raya Puasa
25.12.2006 - Christmas Day - PH in SG
31.12.2006 - Hari Raya Haji - PH in SG
Total: 12 days
26.01.2006 -
14.04.2006 - Good Friday - PH in AU
17.04.2006 - Ester Monday - PH in AU
18.04.2006 - Ester Tuesday - PH in AU
25.04.2006 - Anzac Day - PH in AU
12.06.2006 - Queen's Birthday - PH in AU (except WA)
25.12.2006 - Christmas Day - PH in AU
26.12.2006 - Boxing Day / Proclamation Day - PH in AU
26.01.2006 -
29.01.2006 - Chinese New Year 2557 - PH in ID/MY/SG
30.01.2006 - Chinese New Year - PH in MY/SG
31.01.2006 - Islamic New Year 1427H - PH in ID/MY/SG
30.03.2006 - Hari Raya Nyepi 1928 - Balinese Hindu New Year - PH in ID
11.04.2006 - Prophet Muhammad's Birthday - PH in ID/MY
14.04.2006 - Good Friday - PH in ID/SG/AU
17.04.2006 - Ester Monday - PH in AU
18.04.2006 - Ester Tuesday - PH in AU
25.04.2006 - Anzac Day - PH in AU
01.05.2006 - Labour Day - PH in MY/SG
12.05.2006 - Vasak (Wesak) Day - PH in MY/SG
13.05.2006 - Hari Raya Waisak - PH in ID
25.05.2006 - Ascension Day - PH in ID
03.06.2006 - Birthday of SPB Yang di-Pertuan Agong - PH in MY
12.06.2006 - Queen's Birthday - PH in AU (except WA)
28.07.2006 - SysAdmin Day
09.08.2006 -
17.08.2006 - Indonesian Independence Day - PH in ID
21.08.2006 - Isra Mi'raj Prophet Mohammad SAW - PH in ID
31.08.2006 -
21.10.2006 - Deepavali - PH in SG/MY
23.10.2006 - Cuti Bersama - PH in ID
24.10.2006 - Hari Raya Puasa / Idul Fitri 1427 H - PH in ID/MY/SG
25.10.2006 - Hari Raya Puasa / Idul Fitri 1427 H - PH in ID/MY
26.10.2006 - Cuti Bersama - PH in ID
27.10.2006 - Cuti Bersama - PH in ID
25.12.2006 - Christmas Day - PH in ID/MY/SG/AU
26.12.2006 - Boxing Day / Proclamation Day - PH in AU
31.12.2006 - Hari Raya Haji (Qurban), Idul Adha 1427H - PH in ID/MY/SG
PS: Let me know if you find any mistakes in here.
Posted @ at
1:46 am
What do you think? (there
are 2
comments)